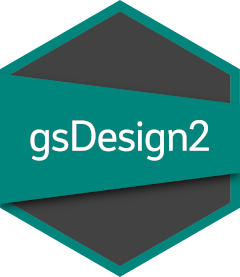
Group sequential design using average hazard ratio under non-proportional hazards
Source:R/gs_update_ahr.R
gs_update_ahr.Rd
Group sequential design using average hazard ratio under non-proportional hazards
Arguments
- x
A design created by either
gs_design_ahr()
orgs_power_ahr()
.- alpha
Type I error for the updated design.
- ustime
Default is NULL in which case upper bound spending time is determined by timing. Otherwise, this should be a vector of length k (total number of analyses) with the spending time at each analysis.
- lstime
Default is NULL in which case lower bound spending time is determined by timing. Otherwise, this should be a vector of length k (total number of analyses) with the spending time at each analysis
- observed_data
a list of observed datasets by analyses.
Examples
library(gsDesign)
library(gsDesign2)
library(dplyr)
alpha <- 0.025
beta <- 0.1
ratio <- 1
# Enrollment
enroll_rate <- define_enroll_rate(
duration = c(2, 2, 10),
rate = (1:3) / 3)
# Failure and dropout
fail_rate <- define_fail_rate(
duration = c(3, Inf), fail_rate = log(2) / 9,
hr = c(1, 0.6), dropout_rate = .0001)
# IA and FA analysis time
analysis_time <- c(20, 36)
# Randomization ratio
ratio <- 1
# ------------------------------------------------- #
# Example A: one-sided design (efficacy only)
# ------------------------------------------------- #
# Original design
upper <- gs_spending_bound
upar <- list(sf = sfLDOF, total_spend = alpha)
x <- gs_design_ahr(
enroll_rate = enroll_rate, fail_rate = fail_rate,
alpha = alpha, beta = beta, ratio = ratio,
info_scale = "h0_info",
info_frac = NULL,
analysis_time = c(20, 36),
upper = gs_spending_bound, upar = upar,
lower = gs_b, lpar = rep(-Inf, 2),
test_upper = TRUE, test_lower = FALSE) |> to_integer()
# Observed dataset at IA and FA
set.seed(123)
observed_data <- simtrial::sim_pw_surv(
n = x$analysis$n[x$analysis$analysis == 2],
stratum = data.frame(stratum = "All", p = 1),
block = c(rep("control", 2), rep("experimental", 2)),
enroll_rate = x$enroll_rate,
fail_rate = (fail_rate |> simtrial::to_sim_pw_surv())$fail_rate,
dropout_rate = (fail_rate |> simtrial::to_sim_pw_surv())$dropout_rate)
observed_data_ia <- observed_data |> simtrial::cut_data_by_date(x$analysis$time[1])
observed_data_fa <- observed_data |> simtrial::cut_data_by_date(x$analysis$time[2])
observed_event_ia <- sum(observed_data_ia$event)
observed_event_fa <- sum(observed_data_fa$event)
planned_event_ia <- x$analysis$event[1]
planned_event_fa <- x$analysis$event[2]
# Example A1 ----
# IA spending = observed events / final planned events
# the remaining alpha will be allocated to FA.
ustime <- c(observed_event_ia / planned_event_fa, 1)
gs_update_ahr(
x = x,
ustime = ustime,
observed_data = list(observed_data_ia, observed_data_fa))
#> $enroll_rate
#> # A tibble: 3 × 3
#> stratum duration rate
#> <chr> <dbl> <dbl>
#> 1 All 2 10.2
#> 2 All 2 20.3
#> 3 All 10 30.5
#>
#> $fail_rate
#> # A tibble: 2 × 5
#> stratum duration fail_rate dropout_rate hr
#> <chr> <dbl> <dbl> <dbl> <dbl>
#> 1 All 3 0.0770 0.0001 1
#> 2 All Inf 0.0770 0.0001 0.6
#>
#> $bound
#> # A tibble: 4 × 7
#> analysis bound probability probability0 z `~hr at bound` `nominal p`
#> <int> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 1 upper 0.315 0.00484 2.59 0.686 0.00484
#> 2 2 upper 0.901 0.0250 1.99 0.794 0.0235
#> 3 1 lower 0 0 -Inf Inf 1
#> 4 2 lower 0 0 -Inf Inf 1
#>
#> $analysis
#> analysis time n event ahr theta info info0 info_frac
#> 1 1 19.96388 366 188 0.7356221 0.3070388 47.00 47.00 0.6329966
#> 2 2 35.81007 366 295 0.6832088 0.3809547 73.75 73.75 1.0000000
#> info_frac0
#> 1 0.6372881
#> 2 1.0000000
#>
#> attr(,"class")
#> [1] "non_binding" "ahr" "gs_design" "list"
#> [5] "updated_design"
# Example A2 ----
# IA, FA spending = observed events / final planned events
ustime <- c(observed_event_ia, observed_event_fa) / planned_event_fa
gs_update_ahr(
x = x,
ustime = ustime,
observed_data = list(observed_data_ia, observed_data_fa))
#> $enroll_rate
#> # A tibble: 3 × 3
#> stratum duration rate
#> <chr> <dbl> <dbl>
#> 1 All 2 10.2
#> 2 All 2 20.3
#> 3 All 10 30.5
#>
#> $fail_rate
#> # A tibble: 2 × 5
#> stratum duration fail_rate dropout_rate hr
#> <chr> <dbl> <dbl> <dbl> <dbl>
#> 1 All 3 0.0770 0.0001 1
#> 2 All Inf 0.0770 0.0001 0.6
#>
#> $bound
#> # A tibble: 4 × 7
#> analysis bound probability probability0 z `~hr at bound` `nominal p`
#> <int> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 1 upper 0.315 0.00484 2.59 0.686 0.00484
#> 2 2 upper 0.899 0.0245 2.00 0.793 0.0230
#> 3 1 lower 0 0 -Inf Inf 1
#> 4 2 lower 0 0 -Inf Inf 1
#>
#> $analysis
#> analysis time n event ahr theta info info0 info_frac
#> 1 1 19.96388 366 188 0.7356221 0.3070388 47.00 47.00 0.6329966
#> 2 2 35.81007 366 295 0.6832088 0.3809547 73.75 73.75 0.9932660
#> info_frac0
#> 1 0.6372881
#> 2 1.0000000
#>
#> attr(,"class")
#> [1] "non_binding" "ahr" "gs_design" "list"
#> [5] "updated_design"
# Example A3 ----
# IA spending = min(observed events, planned events) / final planned events
ustime <- c(min(observed_event_ia, planned_event_ia) / planned_event_fa, 1)
gs_update_ahr(
x = x,
ustime = ustime,
observed_data = list(observed_data_ia, observed_data_fa))
#> $enroll_rate
#> # A tibble: 3 × 3
#> stratum duration rate
#> <chr> <dbl> <dbl>
#> 1 All 2 10.2
#> 2 All 2 20.3
#> 3 All 10 30.5
#>
#> $fail_rate
#> # A tibble: 2 × 5
#> stratum duration fail_rate dropout_rate hr
#> <chr> <dbl> <dbl> <dbl> <dbl>
#> 1 All 3 0.0770 0.0001 1
#> 2 All Inf 0.0770 0.0001 0.6
#>
#> $bound
#> # A tibble: 4 × 7
#> analysis bound probability probability0 z `~hr at bound` `nominal p`
#> <int> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 1 upper 0.315 0.00484 2.59 0.686 0.00484
#> 2 2 upper 0.901 0.0250 1.99 0.794 0.0235
#> 3 1 lower 0 0 -Inf Inf 1
#> 4 2 lower 0 0 -Inf Inf 1
#>
#> $analysis
#> analysis time n event ahr theta info info0 info_frac
#> 1 1 19.96388 366 188 0.7356221 0.3070388 47.00 47.00 0.6329966
#> 2 2 35.81007 366 295 0.6832088 0.3809547 73.75 73.75 1.0000000
#> info_frac0
#> 1 0.6372881
#> 2 1.0000000
#>
#> attr(,"class")
#> [1] "non_binding" "ahr" "gs_design" "list"
#> [5] "updated_design"
# Example A4 ----
# IA spending = min(observed events, planned events) / final planned events
ustime <- c(min(observed_event_ia, planned_event_ia),
min(observed_event_fa, planned_event_fa)) / planned_event_fa
gs_update_ahr(
x = x,
ustime = ustime,
observed_data = list(observed_data_ia, observed_data_fa))
#> $enroll_rate
#> # A tibble: 3 × 3
#> stratum duration rate
#> <chr> <dbl> <dbl>
#> 1 All 2 10.2
#> 2 All 2 20.3
#> 3 All 10 30.5
#>
#> $fail_rate
#> # A tibble: 2 × 5
#> stratum duration fail_rate dropout_rate hr
#> <chr> <dbl> <dbl> <dbl> <dbl>
#> 1 All 3 0.0770 0.0001 1
#> 2 All Inf 0.0770 0.0001 0.6
#>
#> $bound
#> # A tibble: 4 × 7
#> analysis bound probability probability0 z `~hr at bound` `nominal p`
#> <int> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 1 upper 0.315 0.00484 2.59 0.686 0.00484
#> 2 2 upper 0.899 0.0245 2.00 0.793 0.0230
#> 3 1 lower 0 0 -Inf Inf 1
#> 4 2 lower 0 0 -Inf Inf 1
#>
#> $analysis
#> analysis time n event ahr theta info info0 info_frac
#> 1 1 19.96388 366 188 0.7356221 0.3070388 47.00 47.00 0.6329966
#> 2 2 35.81007 366 295 0.6832088 0.3809547 73.75 73.75 0.9932660
#> info_frac0
#> 1 0.6372881
#> 2 1.0000000
#>
#> attr(,"class")
#> [1] "non_binding" "ahr" "gs_design" "list"
#> [5] "updated_design"
# alpha is upadted to 0.05
gs_update_ahr(
x = x,
alpha = 0.05,
ustime = ustime,
observed_data = list(observed_data_ia, observed_data_fa))
#> $enroll_rate
#> # A tibble: 3 × 3
#> stratum duration rate
#> <chr> <dbl> <dbl>
#> 1 All 2 10.2
#> 2 All 2 20.3
#> 3 All 10 30.5
#>
#> $fail_rate
#> # A tibble: 2 × 5
#> stratum duration fail_rate dropout_rate hr
#> <chr> <dbl> <dbl> <dbl> <dbl>
#> 1 All 3 0.0770 0.0001 1
#> 2 All Inf 0.0770 0.0001 0.6
#>
#> $bound
#> # A tibble: 4 × 7
#> analysis bound probability probability0 z `~hr at bound` `nominal p`
#> <int> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 1 upper 0.461 0.0138 2.20 0.725 0.0138
#> 2 2 upper 0.943 0.0492 1.69 0.821 0.0451
#> 3 1 lower 0 0 -Inf Inf 1
#> 4 2 lower 0 0 -Inf Inf 1
#>
#> $analysis
#> analysis time n event ahr theta info info0 info_frac
#> 1 1 19.96388 366 188 0.7356221 0.3070388 47.00 47.00 0.6329966
#> 2 2 35.81007 366 295 0.6832088 0.3809547 73.75 73.75 0.9932660
#> info_frac0
#> 1 0.6372881
#> 2 1.0000000
#>
#> attr(,"class")
#> [1] "non_binding" "ahr" "gs_design" "list"
#> [5] "updated_design"
# ------------------------------------------------- #
# Example B: Two-sided asymmetric design,
# beta-spending with non-binding lower bound
# ------------------------------------------------- #
# Original design
x <- gs_design_ahr(
enroll_rate = enroll_rate, fail_rate = fail_rate,
alpha = alpha, beta = beta, ratio = ratio,
info_scale = "h0_info",
info_frac = NULL, analysis_time = c(20, 36),
upper = gs_spending_bound,
upar = list(sf = sfLDOF, total_spend = alpha),
test_upper = TRUE,
lower = gs_spending_bound,
lpar = list(sf = sfLDOF, total_spend = beta),
test_lower = c(TRUE, FALSE),
binding = FALSE) |> to_integer()
# Example B1 ----
# IA spending = observed events / final planned events
# the remaining alpha will be allocated to FA.
ustime <- c(observed_event_ia / planned_event_fa, 1)
gs_update_ahr(
x = x,
ustime = ustime,
lstime = ustime,
observed_data = list(observed_data_ia, observed_data_fa))
#> $enroll_rate
#> # A tibble: 3 × 3
#> stratum duration rate
#> <chr> <dbl> <dbl>
#> 1 All 2 10.6
#> 2 All 2 21.2
#> 3 All 10 31.8
#>
#> $fail_rate
#> # A tibble: 2 × 5
#> stratum duration fail_rate dropout_rate hr
#> <chr> <dbl> <dbl> <dbl> <dbl>
#> 1 All 3 0.0770 0.0001 1
#> 2 All Inf 0.0770 0.0001 0.6
#>
#> $bound
#> # A tibble: 4 × 7
#> analysis bound probability probability0 z `~hr at bound` `nominal p`
#> <int> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 1 upper 0.315 0.00484 2.59 0.686 0.00484
#> 2 2 upper 0.891 0.0248 1.99 0.794 0.0235
#> 3 1 lower 0.0387 0.633 0.339 0.952 0.367
#> 4 2 lower 0.0387 0.633 -Inf Inf 1
#>
#> $analysis
#> analysis time n event ahr theta info info0 info_frac
#> 1 1 19.91897 382 188 0.7356221 0.3070388 47.00 47.00 0.6329966
#> 2 2 36.06513 382 295 0.6832088 0.3809547 73.75 73.75 1.0000000
#> info_frac0
#> 1 0.6372881
#> 2 1.0000000
#>
#> attr(,"class")
#> [1] "non_binding" "ahr" "gs_design" "list"
#> [5] "updated_design"
# Example B2 ----
# IA, FA spending = observed events / final planned events
ustime <- c(observed_event_ia, observed_event_fa) / planned_event_fa
gs_update_ahr(
x = x,
ustime = ustime,
lstime = ustime,
observed_data = list(observed_data_ia, observed_data_fa))
#> $enroll_rate
#> # A tibble: 3 × 3
#> stratum duration rate
#> <chr> <dbl> <dbl>
#> 1 All 2 10.6
#> 2 All 2 21.2
#> 3 All 10 31.8
#>
#> $fail_rate
#> # A tibble: 2 × 5
#> stratum duration fail_rate dropout_rate hr
#> <chr> <dbl> <dbl> <dbl> <dbl>
#> 1 All 3 0.0770 0.0001 1
#> 2 All Inf 0.0770 0.0001 0.6
#>
#> $bound
#> # A tibble: 4 × 7
#> analysis bound probability probability0 z `~hr at bound` `nominal p`
#> <int> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 1 upper 0.315 0.00484 2.59 0.686 0.00484
#> 2 2 upper 0.890 0.0243 2.00 0.793 0.0230
#> 3 1 lower 0.0387 0.633 0.339 0.952 0.367
#> 4 2 lower 0.0387 0.633 -Inf Inf 1
#>
#> $analysis
#> analysis time n event ahr theta info info0 info_frac
#> 1 1 19.91897 382 188 0.7356221 0.3070388 47.00 47.00 0.6329966
#> 2 2 36.06513 382 295 0.6832088 0.3809547 73.75 73.75 0.9932660
#> info_frac0
#> 1 0.6372881
#> 2 1.0000000
#>
#> attr(,"class")
#> [1] "non_binding" "ahr" "gs_design" "list"
#> [5] "updated_design"
# Example B3 ----
ustime <- c(min(observed_event_ia, planned_event_ia) / planned_event_fa, 1)
gs_update_ahr(
x = x,
ustime = ustime,
lstime = ustime,
observed_data = list(observed_data_ia, observed_data_fa))
#> $enroll_rate
#> # A tibble: 3 × 3
#> stratum duration rate
#> <chr> <dbl> <dbl>
#> 1 All 2 10.6
#> 2 All 2 21.2
#> 3 All 10 31.8
#>
#> $fail_rate
#> # A tibble: 2 × 5
#> stratum duration fail_rate dropout_rate hr
#> <chr> <dbl> <dbl> <dbl> <dbl>
#> 1 All 3 0.0770 0.0001 1
#> 2 All Inf 0.0770 0.0001 0.6
#>
#> $bound
#> # A tibble: 4 × 7
#> analysis bound probability probability0 z `~hr at bound` `nominal p`
#> <int> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 1 upper 0.315 0.00484 2.59 0.686 0.00484
#> 2 2 upper 0.891 0.0248 1.99 0.794 0.0235
#> 3 1 lower 0.0387 0.633 0.339 0.952 0.367
#> 4 2 lower 0.0387 0.633 -Inf Inf 1
#>
#> $analysis
#> analysis time n event ahr theta info info0 info_frac
#> 1 1 19.91897 382 188 0.7356221 0.3070388 47.00 47.00 0.6329966
#> 2 2 36.06513 382 295 0.6832088 0.3809547 73.75 73.75 1.0000000
#> info_frac0
#> 1 0.6372881
#> 2 1.0000000
#>
#> attr(,"class")
#> [1] "non_binding" "ahr" "gs_design" "list"
#> [5] "updated_design"
# Example B4 ----
# IA spending = min(observed events, planned events) / final planned events
ustime <- c(min(observed_event_ia, planned_event_ia),
min(observed_event_fa, planned_event_fa)) / planned_event_fa
gs_update_ahr(
x = x,
ustime = ustime,
lstime = ustime,
observed_data = list(observed_data_ia, observed_data_fa))
#> $enroll_rate
#> # A tibble: 3 × 3
#> stratum duration rate
#> <chr> <dbl> <dbl>
#> 1 All 2 10.6
#> 2 All 2 21.2
#> 3 All 10 31.8
#>
#> $fail_rate
#> # A tibble: 2 × 5
#> stratum duration fail_rate dropout_rate hr
#> <chr> <dbl> <dbl> <dbl> <dbl>
#> 1 All 3 0.0770 0.0001 1
#> 2 All Inf 0.0770 0.0001 0.6
#>
#> $bound
#> # A tibble: 4 × 7
#> analysis bound probability probability0 z `~hr at bound` `nominal p`
#> <int> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 1 upper 0.315 0.00484 2.59 0.686 0.00484
#> 2 2 upper 0.890 0.0243 2.00 0.793 0.0230
#> 3 1 lower 0.0387 0.633 0.339 0.952 0.367
#> 4 2 lower 0.0387 0.633 -Inf Inf 1
#>
#> $analysis
#> analysis time n event ahr theta info info0 info_frac
#> 1 1 19.91897 382 188 0.7356221 0.3070388 47.00 47.00 0.6329966
#> 2 2 36.06513 382 295 0.6832088 0.3809547 73.75 73.75 0.9932660
#> info_frac0
#> 1 0.6372881
#> 2 1.0000000
#>
#> attr(,"class")
#> [1] "non_binding" "ahr" "gs_design" "list"
#> [5] "updated_design"
# Example B5 ----
# alpha is updated to 0.05 ----
gs_update_ahr(x = x, alpha = 0.05)
#> $enroll_rate
#> # A tibble: 3 × 3
#> stratum duration rate
#> <chr> <dbl> <dbl>
#> 1 All 2 10.6
#> 2 All 2 21.2
#> 3 All 10 31.8
#>
#> $fail_rate
#> # A tibble: 2 × 5
#> stratum duration fail_rate dropout_rate hr
#> <chr> <dbl> <dbl> <dbl> <dbl>
#> 1 All 3 0.0770 0.0001 1
#> 2 All Inf 0.0770 0.0001 0.6
#>
#> $bound
#> # A tibble: 4 × 7
#> analysis bound probability probability0 z `~hr at bound` `nominal p`
#> <int> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 1 upper 0.518 0.0150 2.17 0.737 0.0150
#> 2 2 upper 0.933 0.0487 1.69 0.826 0.0455
#> 3 1 lower 0.0413 0.684 0.478 0.935 0.316
#> 4 2 lower 0.0413 0.684 -Inf Inf 1
#>
#> $analysis
#> analysis time n event ahr theta info info0 info_frac
#> 1 1 19.91897 382 202 0.7322996 0.3115656 50.50 50.50 0.6495177
#> 2 2 36.06513 382 311 0.6829028 0.3814027 77.75 77.75 1.0000000
#> info_frac0
#> 1 0.6495177
#> 2 1.0000000
#>
#> attr(,"class")
#> [1] "non_binding" "ahr" "gs_design" "list"
#> [5] "updated_design"
# Example B6 ----
# updated boundaries only when IA data is observed
ustime <- c(observed_event_ia / planned_event_fa, 1)
gs_update_ahr(
x = x,
ustime = ustime,
lstime = ustime,
observed_data = list(observed_data_ia, NULL))
#> $enroll_rate
#> # A tibble: 3 × 3
#> stratum duration rate
#> <chr> <dbl> <dbl>
#> 1 All 2 10.6
#> 2 All 2 21.2
#> 3 All 10 31.8
#>
#> $fail_rate
#> # A tibble: 2 × 5
#> stratum duration fail_rate dropout_rate hr
#> <chr> <dbl> <dbl> <dbl> <dbl>
#> 1 All 3 0.0770 0.0001 1
#> 2 All Inf 0.0770 0.0001 0.6
#>
#> $bound
#> # A tibble: 4 × 7
#> analysis bound probability probability0 z `~hr at bound` `nominal p`
#> <int> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 1 upper 0.315 0.00484 2.59 0.686 0.00484
#> 2 2 upper 0.903 0.0247 1.99 0.798 0.0233
#> 3 1 lower 0.0387 0.633 0.339 0.952 0.367
#> 4 2 lower 0.0387 0.633 -Inf Inf 1
#>
#> $analysis
#> analysis time n event ahr theta info info0 info_frac
#> 1 1 19.91897 382 188 0.7356221 0.3070388 47.00 47.00 0.6329966
#> 2 2 36.06513 382 311 0.6829028 0.3814027 77.75 77.75 1.0000000
#> info_frac0
#> 1 0.6045016
#> 2 1.0000000
#>
#> attr(,"class")
#> [1] "non_binding" "ahr" "gs_design" "list"
#> [5] "updated_design"
# ------------------------------------------------- #
# Example C: Two-sided asymmetric design,
# with calendar spending for efficacy and futility bounds
# beta-spending with non-binding lower bound
# ------------------------------------------------- #
# Original design
x <- gs_design_ahr(
enroll_rate = enroll_rate, fail_rate = fail_rate,
alpha = alpha, beta = beta, ratio = ratio,
info_scale = "h0_info",
info_frac = NULL, analysis_time = c(20, 36),
upper = gs_spending_bound,
upar = list(sf = sfLDOF, total_spend = alpha, timing = c(20, 36) / 36),
test_upper = TRUE,
lower = gs_spending_bound,
lpar = list(sf = sfLDOF, total_spend = beta, timing = c(20, 36) / 36),
test_lower = c(TRUE, FALSE),
binding = FALSE) |> to_integer()
# Updated design due to potential change of multiplicity graph
gs_update_ahr(x = x, alpha = 0.05)
#> $enroll_rate
#> # A tibble: 3 × 3
#> stratum duration rate
#> <chr> <dbl> <dbl>
#> 1 All 2 10.2
#> 2 All 2 20.4
#> 3 All 10 30.7
#>
#> $fail_rate
#> # A tibble: 2 × 5
#> stratum duration fail_rate dropout_rate hr
#> <chr> <dbl> <dbl> <dbl> <dbl>
#> 1 All 3 0.0770 0.0001 1
#> 2 All Inf 0.0770 0.0001 0.6
#>
#> $bound
#> # A tibble: 4 × 7
#> analysis bound probability probability0 z `~hr at bound` `nominal p`
#> <int> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 1 upper 0.418 0.00855 2.38 0.711 0.00855
#> 2 2 upper 0.940 0.0493 1.66 0.825 0.0483
#> 3 1 lower 0.0273 0.601 0.257 0.964 0.399
#> 4 2 lower 0.0273 0.601 -Inf Inf 1
#>
#> $analysis
#> analysis time n event ahr theta info info0 info_frac
#> 1 1 19.95805 368 195 0.7319980 0.3119776 48.75 48.75 0.65
#> 2 2 36.16991 368 300 0.6827856 0.3815744 75.00 75.00 1.00
#> info_frac0
#> 1 0.65
#> 2 1.00
#>
#> attr(,"class")
#> [1] "non_binding" "ahr" "gs_design" "list"
#> [5] "updated_design"