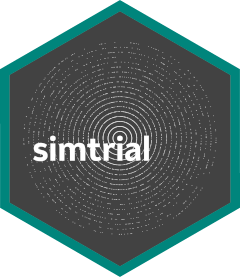
Simulation of fixed sample size design for time-to-event endpoint
Source:R/sim_fixed_n.R
sim_fixed_n.Rd
sim_fixed_n()
provides simulations of a single endpoint two-arm trial
where the enrollment, hazard ratio, and failure and dropout rates change
over time.
Usage
sim_fixed_n(
n_sim = 1000,
sample_size = 500,
target_event = 350,
stratum = data.frame(stratum = "All", p = 1),
enroll_rate = data.frame(duration = c(2, 2, 10), rate = c(3, 6, 9)),
fail_rate = data.frame(stratum = "All", duration = c(3, 100), fail_rate = log(2)/c(9,
18), hr = c(0.9, 0.6), dropout_rate = rep(0.001, 2)),
total_duration = 30,
block = rep(c("experimental", "control"), 2),
timing_type = 1:5,
rho_gamma = data.frame(rho = 0, gamma = 0)
)
Arguments
- n_sim
Number of simulations to perform.
- sample_size
Total sample size per simulation.
- target_event
Targeted event count for analysis.
- stratum
A data frame with stratum specified in
stratum
, probability (incidence) of each stratum inp
.- enroll_rate
Piecewise constant enrollment rates by time period. Note that these are overall population enrollment rates and the
stratum
argument controls the random distribution between stratum.- fail_rate
Piecewise constant control group failure rates, hazard ratio for experimental vs. control, and dropout rates by stratum and time period.
- total_duration
Total follow-up from start of enrollment to data cutoff.
- block
As in
sim_pw_surv()
. Vector of treatments to be included in each block.- timing_type
A numeric vector determining data cutoffs used; see details. Default is to include all available cutoff methods.
- rho_gamma
A data frame with variables
rho
andgamma
, both greater than equal to zero, to specify one Fleming-Harrington weighted logrank test per row.
Value
A data frame including columns:
event
: Event count.ln_hr
: Log-hazard ratio.z
: Normal test statistic; < 0 favors experimental.cut
: Text describing cutoff used.duration
: Duration of trial at cutoff for analysis.sim
: Sequential simulation ID.
One row per simulated dataset per cutoff specified in timing_type
,
per test statistic specified.
If multiple Fleming-Harrington tests are specified in rho_gamma
,
then columns rho
and gamma
are also included.
Details
timing_type
has up to 5 elements indicating different options
for data cutoff:
1
: Uses the planned study duration.2
: The time the targeted event count is achieved.3
: The planned minimum follow-up after enrollment is complete.4
: The maximum of planned study duration and targeted event count cuts (1 and 2).5
: The maximum of targeted event count and minimum follow-up cuts (2 and 3).
Examples
library(dplyr)
library(future)
# Example 1: logrank test ----
x <- sim_fixed_n(n_sim = 10, timing_type = 1, rho_gamma = data.frame(rho = 0, gamma = 0))
#> Backend uses sequential processing.
# Get power approximation
mean(x$z <= qnorm(.025))
#> [1] 0
# Example 2: WLR with FH(0,1) ----
sim_fixed_n(n_sim = 1, timing_type = 1, rho_gamma = data.frame(rho = 0, gamma = 1))
#> Backend uses sequential processing.
#> method parameter estimate se z event ln_hr
#> 1 WLR FH(rho=0, gamma=1) -1.608741 1.14817 1.401136 90 -0.2204257
#> cut duration sim
#> 1 Planned duration 30 1
# Get power approximation
mean(x$z <= qnorm(.025))
#> [1] 0
# \donttest{
# Example 3: MaxCombo, i.e., WLR-FH(0,0)+ WLR-FH(0,1)
# Power by test
# Only use cuts for events, events + min follow-up
x <- sim_fixed_n(
n_sim = 10,
timing_type = 2,
rho_gamma = data.frame(rho = 0, gamma = c(0, 1))
)
#> Backend uses sequential processing.
# Get power approximation
x |>
group_by(sim) |>
filter(row_number() == 1) |>
ungroup() |>
summarize(power = mean(p_value < .025))
#> # A tibble: 1 × 1
#> power
#> <dbl>
#> 1 1
# Example 4
# Use two cores
set.seed(2023)
plan("multisession", workers = 2)
sim_fixed_n(n_sim = 10)
#> Using 2 cores with backend multisession
#> method parameter estimate se z event ln_hr
#> 1 WLR FH(rho=0, gamma=0) -9.044800 5.213492 1.7348832 109 -0.3342849
#> 2 WLR FH(rho=0, gamma=0) -41.170785 9.229540 4.4607622 350 -0.4793575
#> 3 WLR FH(rho=0, gamma=0) -41.444949 9.546994 4.3411518 376 -0.4498374
#> 4 WLR FH(rho=0, gamma=0) -41.170785 9.229540 4.4607622 350 -0.4793575
#> 5 WLR FH(rho=0, gamma=0) -41.444949 9.546994 4.3411518 376 -0.4498374
#> 6 WLR FH(rho=0, gamma=0) -5.248725 5.068148 1.0356297 103 -0.2047494
#> 7 WLR FH(rho=0, gamma=0) -33.266167 9.245312 3.5981660 350 -0.3873648
#> 8 WLR FH(rho=0, gamma=0) -35.663072 9.468334 3.7665623 367 -0.3953215
#> 9 WLR FH(rho=0, gamma=0) -33.266167 9.245312 3.5981660 350 -0.3873648
#> 10 WLR FH(rho=0, gamma=0) -35.663072 9.468334 3.7665623 367 -0.3953215
#> 11 WLR FH(rho=0, gamma=0) -18.821290 4.838510 3.8898935 95 -0.8181040
#> 12 WLR FH(rho=0, gamma=0) -55.442685 9.055033 6.1228581 350 -0.6662357
#> 13 WLR FH(rho=0, gamma=0) -55.753830 9.043216 6.1652657 349 -0.6719858
#> 14 WLR FH(rho=0, gamma=0) -55.442685 9.055033 6.1228581 350 -0.6662357
#> 15 WLR FH(rho=0, gamma=0) -55.442685 9.055033 6.1228581 350 -0.6662357
#> 16 WLR FH(rho=0, gamma=0) -3.064636 5.228765 0.5861109 110 -0.1120785
#> 17 WLR FH(rho=0, gamma=0) -33.961737 9.264232 3.6658988 350 -0.3939024
#> 18 WLR FH(rho=0, gamma=0) -34.607774 9.536543 3.6289642 372 -0.3781111
#> 19 WLR FH(rho=0, gamma=0) -33.961737 9.264232 3.6658988 350 -0.3939024
#> 20 WLR FH(rho=0, gamma=0) -34.607774 9.536543 3.6289642 372 -0.3781111
#> 21 WLR FH(rho=0, gamma=0) -13.960942 4.823458 2.8943845 94 -0.6069906
#> 22 WLR FH(rho=0, gamma=0) -37.851426 9.213540 4.1082391 350 -0.4410650
#> 23 WLR FH(rho=0, gamma=0) -36.640901 9.184545 3.9894083 348 -0.4296748
#> 24 WLR FH(rho=0, gamma=0) -37.851426 9.213540 4.1082391 350 -0.4410650
#> 25 WLR FH(rho=0, gamma=0) -37.851426 9.213540 4.1082391 350 -0.4410650
#> 26 WLR FH(rho=0, gamma=0) -14.173675 5.030231 2.8176983 103 -0.5638412
#> 27 WLR FH(rho=0, gamma=0) -35.792430 9.171024 3.9027737 350 -0.4227156
#> 28 WLR FH(rho=0, gamma=0) -36.093884 9.190638 3.9272445 351 -0.4245379
#> 29 WLR FH(rho=0, gamma=0) -35.792430 9.171024 3.9027737 350 -0.4227156
#> 30 WLR FH(rho=0, gamma=0) -36.093884 9.190638 3.9272445 351 -0.4245379
#> 31 WLR FH(rho=0, gamma=0) -4.479885 5.189040 0.8633360 108 -0.1666295
#> 32 WLR FH(rho=0, gamma=0) -29.984429 9.309267 3.2209226 350 -0.3453421
#> 33 WLR FH(rho=0, gamma=0) -27.732193 9.152805 3.0299119 338 -0.3305844
#> 34 WLR FH(rho=0, gamma=0) -29.984429 9.309267 3.2209226 350 -0.3453421
#> 35 WLR FH(rho=0, gamma=0) -29.984429 9.309267 3.2209226 350 -0.3453421
#> 36 WLR FH(rho=0, gamma=0) -8.620094 5.553024 1.5523243 124 -0.2803523
#> 37 WLR FH(rho=0, gamma=0) -30.211007 9.300647 3.2482694 350 -0.3478454
#> 38 WLR FH(rho=0, gamma=0) -33.581256 9.516244 3.5288351 367 -0.3690357
#> 39 WLR FH(rho=0, gamma=0) -30.211007 9.300647 3.2482694 350 -0.3478454
#> 40 WLR FH(rho=0, gamma=0) -33.581256 9.516244 3.5288351 367 -0.3690357
#> 41 WLR FH(rho=0, gamma=0) -3.955284 5.093447 0.7765436 104 -0.1525416
#> 42 WLR FH(rho=0, gamma=0) -26.670986 9.302656 2.8670291 350 -0.3069406
#> 43 WLR FH(rho=0, gamma=0) -26.342504 9.314768 2.8280363 351 -0.3023229
#> 44 WLR FH(rho=0, gamma=0) -26.670986 9.302656 2.8670291 350 -0.3069406
#> 45 WLR FH(rho=0, gamma=0) -26.342504 9.314768 2.8280363 351 -0.3023229
#> 46 WLR FH(rho=0, gamma=0) -9.242625 5.104900 1.8105400 106 -0.3551148
#> 47 WLR FH(rho=0, gamma=0) -35.255531 9.270632 3.8029263 350 -0.4077490
#> 48 WLR FH(rho=0, gamma=0) -36.706949 9.386743 3.9105095 360 -0.4138911
#> 49 WLR FH(rho=0, gamma=0) -35.255531 9.270632 3.8029263 350 -0.4077490
#> 50 WLR FH(rho=0, gamma=0) -36.706949 9.386743 3.9105095 360 -0.4138911
#> cut duration sim
#> 1 Planned duration 30.00000 1
#> 2 Targeted events 65.23187 1
#> 3 Minimum follow-up 73.17268 1
#> 4 Max(planned duration, event cut) 65.23187 1
#> 5 Max(min follow-up, event cut) 73.17268 1
#> 6 Planned duration 30.00000 2
#> 7 Targeted events 67.64612 2
#> 8 Minimum follow-up 72.56793 2
#> 9 Max(planned duration, event cut) 67.64612 2
#> 10 Max(min follow-up, event cut) 72.56793 2
#> 11 Planned duration 30.00000 3
#> 12 Targeted events 74.95821 3
#> 13 Minimum follow-up 74.72225 3
#> 14 Max(planned duration, event cut) 74.95821 3
#> 15 Max(min follow-up, event cut) 74.95821 3
#> 16 Planned duration 30.00000 4
#> 17 Targeted events 69.16631 4
#> 18 Minimum follow-up 73.68989 4
#> 19 Max(planned duration, event cut) 69.16631 4
#> 20 Max(min follow-up, event cut) 73.68989 4
#> 21 Planned duration 30.00000 5
#> 22 Targeted events 77.05035 5
#> 23 Minimum follow-up 76.68470 5
#> 24 Max(planned duration, event cut) 77.05035 5
#> 25 Max(min follow-up, event cut) 77.05035 5
#> 26 Planned duration 30.00000 6
#> 27 Targeted events 71.51268 6
#> 28 Minimum follow-up 72.34254 6
#> 29 Max(planned duration, event cut) 71.51268 6
#> 30 Max(min follow-up, event cut) 72.34254 6
#> 31 Planned duration 30.00000 7
#> 32 Targeted events 75.61250 7
#> 33 Minimum follow-up 72.99192 7
#> 34 Max(planned duration, event cut) 75.61250 7
#> 35 Max(min follow-up, event cut) 75.61250 7
#> 36 Planned duration 30.00000 8
#> 37 Targeted events 66.62160 8
#> 38 Minimum follow-up 70.19488 8
#> 39 Max(planned duration, event cut) 66.62160 8
#> 40 Max(min follow-up, event cut) 70.19488 8
#> 41 Planned duration 30.00000 9
#> 42 Targeted events 70.92746 9
#> 43 Minimum follow-up 71.28078 9
#> 44 Max(planned duration, event cut) 70.92746 9
#> 45 Max(min follow-up, event cut) 71.28078 9
#> 46 Planned duration 30.00000 10
#> 47 Targeted events 75.17856 10
#> 48 Minimum follow-up 76.94235 10
#> 49 Max(planned duration, event cut) 75.17856 10
#> 50 Max(min follow-up, event cut) 76.94235 10
plan("sequential")
# }