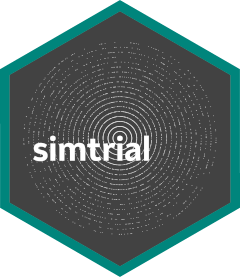
Derive analysis date for interim/final analysis given multiple conditions
Source:R/get_analysis_date.R
get_analysis_date.Rd
Derive analysis date for interim/final analysis given multiple conditions
Usage
get_analysis_date(
data,
planned_calendar_time = NA,
target_event_overall = NA,
target_event_per_stratum = NA,
max_extension_for_target_event = NA,
previous_analysis_date = 0,
min_time_after_previous_analysis = NA,
min_n_overall = NA,
min_n_per_stratum = NA,
min_followup = NA
)
Arguments
- data
A simulated data generated by
sim_pw_surv()
.- planned_calendar_time
A numerical value specifying the planned calendar time for the analysis.
- target_event_overall
A numerical value specifying the targeted events for the overall population.
- target_event_per_stratum
A numerical vector specifying the targeted events per stratum.
- max_extension_for_target_event
A numerical value specifying the maximum time extension to reach targeted events.
- previous_analysis_date
A numerical value specifying the previous analysis date.
- min_time_after_previous_analysis
A numerical value specifying the planned minimum time after the previous analysis.
- min_n_overall
A numerical value specifying the minimal overall sample size enrolled to kick off the analysis.
- min_n_per_stratum
A numerical value specifying the minimal sample size enrolled per stratum to kick off the analysis.
- min_followup
A numerical value specifying the minimal follow-up time after specified enrollment fraction in
min_n_overall
ormin_n_per_stratum
.
Details
To obtain the analysis date, consider the following multiple conditions:
- Condition 1
The planned calendar time for analysis.
- Condition 2
The targeted events, encompassing both overall population and stratum-specific events.
- Condition 3
The maximum time extension required to achieve the targeted events.
- Condition 4
The planned minimum time interval after the previous analysis.
- Condition 5
The minimum follow-up time needed to reach a certain number of patients in enrollments.
Users have the flexibility to employ all 5 conditions simultaneously or
selectively choose specific conditions to determine the analysis date.
Any unused conditions will default to NA
and not affect the output.
Regardless of the number of conditions used, the analysis date is determined
by min(max(date1, date2, date4, date5, na.rm = TRUE), date3, na.rm = TRUE)
,
where date1
, date2
, date3
, date4
, date5
represent the analysis
dates determined solely by Condition 1, Condition 2, Condition 3,
Condition 4 and Condition 5, respectively.
Examples
library(gsDesign2)
alpha <- 0.025
ratio <- 3
n <- 500
info_frac <- c(0.7, 1)
prevalence_ratio <- c(0.4, 0.6)
study_duration <- 48
# Two strata
stratum <- c("Biomarker-positive", "Biomarker-negative")
prevalence_ratio <- c(0.6, 0.4)
# enrollment rate
enroll_rate <- define_enroll_rate(
stratum = rep(stratum, each = 2),
duration = c(2, 10, 2, 10),
rate = c(c(1, 4) * prevalence_ratio[1], c(1, 4) * prevalence_ratio[2])
)
enroll_rate$rate <- enroll_rate$rate * n / sum(enroll_rate$duration * enroll_rate$rate)
# Failure rate
med_pos <- 10 # Median of the biomarker positive population
med_neg <- 8 # Median of the biomarker negative population
hr_pos <- c(1, 0.7) # Hazard ratio of the biomarker positive population
hr_neg <- c(1, 0.8) # Hazard ratio of the biomarker negative population
fail_rate <- define_fail_rate(
stratum = rep(stratum, each = 2),
duration = 1000,
fail_rate = c(log(2) / c(med_pos, med_pos, med_neg, med_neg)),
hr = c(hr_pos, hr_neg),
dropout_rate = 0.01
)
# Simulate data
temp <- to_sim_pw_surv(fail_rate) # Convert the failure rate
set.seed(2023)
simulated_data <- sim_pw_surv(
n = n, # Sample size
# Stratified design with prevalence ratio of 6:4
stratum = data.frame(stratum = stratum, p = prevalence_ratio),
# Randomization ratio
block = c("control", "control", "experimental", "experimental"),
enroll_rate = enroll_rate, # Enrollment rate
fail_rate = temp$fail_rate, # Failure rate
dropout_rate = temp$dropout_rate # Dropout rate
)
# Example 1: Cut for analysis at the 24th month.
# Here, we only utilize the `planned_calendar_time = 24` argument,
# while leaving the remaining unused arguments as their default value of `NA`.
get_analysis_date(
simulated_data,
planned_calendar_time = 24
)
#> [1] 24
# Example 2: Cut for analysis when there are 300 events in the overall population.
# Here, we only utilize the `target_event_overall = 300` argument,
# while leaving the remaining unused arguments as their default value of `NA`.
get_analysis_date(
simulated_data,
target_event_overall = 300
)
#> [1] 25.61506
# Example 3: Cut for analysis at the 24th month and there are 300 events
# in the overall population, whichever arrives later.
# Here, we only utilize the `planned_calendar_time = 24` and
# `target_event_overall = 300` argument,
# while leaving the remaining unused arguments as their default value of `NA`.
get_analysis_date(
simulated_data,
planned_calendar_time = 24,
target_event_overall = 300
)
#> [1] 25.61506
# Example 4a: Cut for analysis when there are at least 100 events
# in the biomarker-positive population, and at least 200 events
# in the biomarker-negative population, whichever arrives later.
# Here, we only utilize the `target_event_per_stratum = c(100, 200)`,
# which refers to 100 events in the biomarker-positive population,
# and 200 events in the biomarker-negative population.
# The remaining unused arguments as their default value of `NA`,
# so the analysis date is only decided by the number of events
# in each stratum.
get_analysis_date(
simulated_data,
target_event_per_stratum = c(100, 200)
)
#> [1] 30.78865
# Example 4b: Cut for analysis when there are at least 100 events
# in the biomarker-positive population, but we don't have a requirement
# for the biomarker-negative population. Additionally, we want to cut
# the analysis when there are at least 150 events in total.
# Here, we only utilize the `target_event_overall = 150` and
# `target_event_per_stratum = c(100, NA)`, which refers to 100 events
# in the biomarker-positive population, and there is event requirement
# for the biomarker-negative population.
# The remaining unused arguments as their default value of `NA`,
# so the analysis date is only decided by the number of events
# in the biomarker-positive population, and the total number of events,
# which arrives later.
get_analysis_date(
simulated_data,
target_event_overall = 150,
target_event_per_stratum = c(100, NA)
)
#> [1] 18.30272
# Example 4c: Cut for analysis when there are at least 100 events
# in the biomarker-positive population, but we don't have a requirement
# for the biomarker-negative population. Additionally, we want to cut
# the analysis when there are at least 150 events in total and after 24 months.
# Here, we only utilize the `planned_calendar_time = 24`,
# `target_event_overall = 150` and
# `target_event_per_stratum = c(100, NA)`, which refers to 100 events
# in the biomarker-positive population, and there is event requirement
# for the biomarker-negative population.
# The remaining unused arguments as their default value of `NA`,
# so the analysis date is only decided by the number of events
# in the biomarker-positive population, the total number of events, and
# planned calendar time, which arrives later.
get_analysis_date(
simulated_data,
planned_calendar_time = 24,
target_event_overall = 150,
target_event_per_stratum = c(100, NA)
)
#> [1] 24
# Example 5: Cut for analysis when there are at least 100 events
# in the biomarker positive population, and at least 200 events
# in the biomarker negative population, whichever arrives later.
# But will stop at the 30th month if events are fewer than 100/200.
# Here, we only utilize the `max_extension_for_target_event = 30`,
# and `target_event_per_stratum = c(100, 200)`, which refers to
# 100/200 events in the biomarker-positive/negative population.
# The remaining unused arguments as their default value of `NA`,
# so the analysis date is only decided by the number of events
# in the 2 strata, and the max extension to arrive at the targeted
# events, which arrives later.
get_analysis_date(
simulated_data,
target_event_per_stratum = c(100, 200),
max_extension_for_target_event = 30
)
#> [1] 30
# Example 6a: Cut for analysis after 12 months followup when 80%
# of the patients are enrolled in the overall population.
# The remaining unused arguments as their default value of `NA`,
# so the analysis date is only decided by
# 12 months + time when 80% patients enrolled.
get_analysis_date(
simulated_data,
min_n_overall = n * 0.8,
min_followup = 12
)
#> [1] 28.82521
# Example 6b: Cut for analysis after 12 months followup when 80%
# of the patients are enrolled in the overall population. Besides,
# the analysis happens when there are at least 150 events in total.
# The remaining unused arguments as their default value of `NA`,
# so the analysis date is only decided by the total number of events,
# and 12 months + time when 80% patients enrolled, which arrives later.
get_analysis_date(
simulated_data,
target_event_overall = 150,
min_n_overall = n * 0.8,
min_followup = 12
)
#> [1] 28.82521
# Example 7a: Cut for analysis when 12 months after at least 200/160 patients
# are enrolled in the biomarker positive/negative population.
# The remaining unused arguments as their default value of `NA`,
# so the analysis date is only decided by 12 months + time when there are
# 200/160 patients enrolled in the biomarker-positive/negative stratum.
get_analysis_date(
simulated_data,
min_n_per_stratum = c(200, 160),
min_followup = 12
)
#> [1] 27.33728
# Example 7b: Cut for analysis when 12 months after at least 200 patients
# are enrolled in the biomarker positive population, but we don't have a
# specific requirement for the biomarker negative population.
# The remaining unused arguments as their default value of `NA`,
# so the analysis date is only decided by 12 months + time when there are
# 200 patients enrolled in the biomarker-positive stratum.
get_analysis_date(
simulated_data,
min_n_per_stratum = c(200, NA),
min_followup = 12
)
#> [1] 27.33728
# Example 7c: Cut for analysis when 12 months after at least 200 patients
# are enrolled in the biomarker-positive population, but we don't have a
# specific requirement for the biomarker-negative population. We also want
# there are at least 80% of the patients enrolled in the overall population.
# The remaining unused arguments as their default value of `NA`,
# so the analysis date is only decided by 12 months + max(time when there are
# 200 patients enrolled in the biomarker-positive stratum, time when there are
# 80% patients enrolled).
get_analysis_date(
simulated_data,
min_n_overall = n * 0.8,
min_n_per_stratum = c(200, NA),
min_followup = 12
)
#> [1] 28.82521